Giới thiệu :
Đối với các ngôn ngữ lập trình khác như C++ , ta đều có thể truy xuất đến các regedit của windows , thông qua đó ta có thể chỉnh sữa , update , tạo mới chúng . Ngoài ra , ta có thể xóa regedit .
Ứng dụng của registry trong C# :
Ta có thể sửa trực tiếp registry mà virus đã làm ẩn một số quyền của máy , hoặc thay đổi thông số của windows .
Vậy làm thế nào để có thể thực hiện những điều đó trong C# ?
Trong C# , điều này rất dễ thực hiện vì C# đã cung cấp thư viện có sẵn để thực hiện . Ta cần sử dụng namespace Microsoft.Win32 .
Trong namespace này sẽ cung cấp cho ta các thuộc tính và phương thức để có thể sử dụng registry .
Ta khai báo như sau :
RegistryKey rk = RegistryKey.[HK cần sử dụng];
vd : ReistryKey rk= RegistryKey.CurrentUser;
Để truy xuất đến subkey cần xử lý key ta thực hiện như sau :
RegistryKey nk = rk.OpenSubKey(đường dẫn)
vd : RegistryKey sk1 = rk.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Policies\\System", true);
Trong đó true là quyền được chỉnh sữa tại subkey này .
Để thay đổi giá trị thì sử dụng hàm SetValue(tên_key,giá_trị)
vd : sk1.SetValue( "DisableTaskMgr",1);
Để lấy giá trị của 1 key : GetValue(tên_key)
Một số key cần thiết trong Regitry
Ẩn/Hiện TaskManger :
CurrentUser\Software\Microsoft\Windows\CurrentVersion\Policies\System\DisableTaskMgr 1-tắt 0-mở .
Ẩn/Hiện RegistryTools :
CurrentUser\Software\Microsoft\Windows\CurrentVersion\Policies\System\DisableRegistryTools 1-tắt 0-mở
Ẩn/Hiện lựa chọn Show file ẩn :
LocalMachine\Software\Microsoft\Windows\CurrentVersion\Explorer\Advanced\Folder\Hidden\SHOWALL\CheckedValue
Khởi động cùng win :
LocalMachine\Software\Microsoft\Windows\CurrentVersion\Run\("tên") giá trị : đường dẫn
Sẽ còn tiếp tục update .
Updating ....
Tổng số lượt xem trang
Thứ Sáu, 31 tháng 12, 2010
Thứ Ba, 28 tháng 12, 2010
Hooking C#
Hook là gì ?
Hook là một kỹ thuật dùng để chặn các sự kiện trước khi chúng được đưa vào stack của ứng dụng . Khi sử dụng kỹ thuật này ta chặn các sự kiện lại và xử lý chúng theo nhu cầu của ta .Ưu điểm ?
Có thể nói hook có ưu điểm rất lớn , ta có thể sử dụng hook để bắt các sự kiện keyboard , mouse ,... Một số chương trình sử dụng hook như Unikey , autogame , trojan ,... Ngoài ra, ta có thể thay đổi giao diện của ứng dụng .Phân loại :
Hook gồm 2 loại chính đó là :Hook cục bộ . Đây là loại hook được móc vào một ứng dụng nhất định .
Hook toàn cục . Đây là hook dùng để móc vào hệ thống thu nhận các sự kiện , thông điệp của toàn hệ thống , bao gồm chương trình .
Hoạt động của một hook ?
Đăng kí hook bằng hàm SetWindowsHookEx với tham số truyền vào là loại hook (mouse,keyboard) , con trỏ hàm xử lý hook , vùng hook (toàn cục, cục bộ) .Thực hiện xử lý hook CallBack .
Hủy hook bằng hàm UnhookWindowsHookEx .
Làm thế nào để cài đặt 1 hook ?
Ở bài này , ta sẽ thực hiện cài đặt một hook chặn thông điệp bàn phím . Từ đó , ta có thể ghi nhận tất cả những phím được gõ , sau đó xuất lên cửa sổ command Dos .Bước 1 : Tạo project Console Application.
Bước 2 : Tạo class Hook .
Ở bước này ta khai báo các thư viện :
using System;
using System.Diagnostics;
using System.Windows.Forms;
using System.Runtime.InteropServices;
Tiếp theo ta Import các DLL để lấy các hàm SetWindowsHookEx ,UnhookWindowsHookEx ,CallNextHookEx ,GetModule .
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
private static extern IntPtr SetWindowsHookEx(int idHook,
LowLevelKeyboardProc lpfn, IntPtr hMod, uint dwThreadId);
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
[return: MarshalAs(UnmanagedType.Bool)]
public static extern bool UnhookWindowsHookEx(IntPtr hhk);
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
private static extern IntPtr CallNextHookEx(IntPtr hhk, int nCode,
IntPtr wParam, IntPtr lParam);
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
private static extern IntPtr GetModuleHandle(string lpModuleName);
private delegate IntPtr LowLevelKeyboardProc(
int nCode, IntPtr wParam, IntPtr lParam);
Khai báo các hàng số và biến
private const int WH_KEYBOARD_LL = 13;
private const int WM_KEYDOWN = 0x0100;
private static LowLevelKeyboardProc _proc = HookCallback;
private static IntPtr _hookID = IntPtr.Zero;
Thiết lập hàm hook
private static IntPtr SetHook(LowLevelKeyboardProc proc)
{
using (Process curProcess = Process.GetCurrentProcess())
using (ProcessModule curModule = curProcess.MainModule)
{
return SetWindowsHookEx(WH_KEYBOARD_LL, proc,
GetModuleHandle(curModule.ModuleName), 0);
}
}
Thiết lập hàm xử lý khi chặn các sự kiện
private static IntPtr HookCallback(
int nCode, IntPtr wParam, IntPtr lParam)
{
if (nCode >= 0 && wParam == (IntPtr)WM_KEYDOWN)
{
int vkCode = Marshal.ReadInt32(lParam);
Console.WriteLine((Keys)vkCode);
}
return CallNextHookEx(_hookID, nCode, wParam, lParam);
}
Vì thế Constructor của hook sẽ là
public Hook()
{
_hookID = SetHook(_proc);
}
Bước 3 : Cài đặt hook ở chương trình
static void main()
{
hook = new Hook();
Application.Run();
}
Chủ Nhật, 19 tháng 12, 2010
Kinect - New future
Do you like game ?
Do you want to fit your body ?
Why dont u use Kinect ? It's product of Microsoft . You can connect Kinect with XBOX 360
Kinect is a new recognized technology , it can connect with XBOX 360 , so you can play game XBOX 360 through Kinect .Kinect use a camera to recognize your movement . And then it will display on the screen . By using Kinect , you will control your action on XBOX 360 .
The player can some action like run , beat , jump and so on ... Because of camera , so you dont need to use remote like Wii or PlayStation Move . It's called free handle when you play game . And you'll feel very exciting about the game and absolutely enjoy your game . You will not bother about broken glass/TV ,....
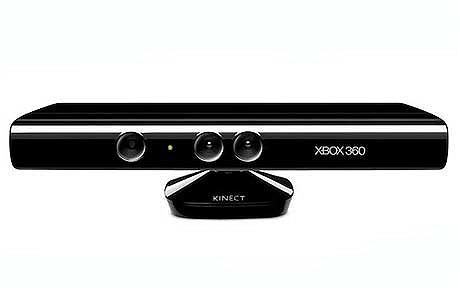
Beside that , Kinect has speech recognize , it can recognize your voice (English only) . Kinect has 4 equipment to recognize accurately . Thus , you can record the voice well , it will reduce interference .
Kinect can recognize than 48 movement of player , and beside that it can be used for multiplayer . 2 players can play within Kinect . This is power of Kinect .
[youtube=http://www.youtube.com/v/iK_UlfO42sc?fs=1&]
The equipment is small and it's easy to take when you go outsite . You can take Kinect when you go to picnic .
Let play Kinect and feel by yourseft . It become real .
How to make money online - PTC ?
My proof of payments : *just one of many*
1/ From Onbux : http://goo.gl/XysNN
2/ From Neobux : http://goo.gl/8HTns
3/ From IncraseBux : http://goo.gl/6MWNa
Updating ...

AND :

AND :
AND :
AND :


AND :

AND :

For more information : http://ptc-investigation.com/
Happy Earning :-)
1/ From Onbux : http://goo.gl/XysNN
2/ From Neobux : http://goo.gl/8HTns
3/ From IncraseBux : http://goo.gl/6MWNa
Updating ...

AND :

AND :

AND :

AND :

AND :

AND :

For more information : http://ptc-investigation.com/
Happy Earning :-)
Đăng ký:
Bài đăng (Atom)